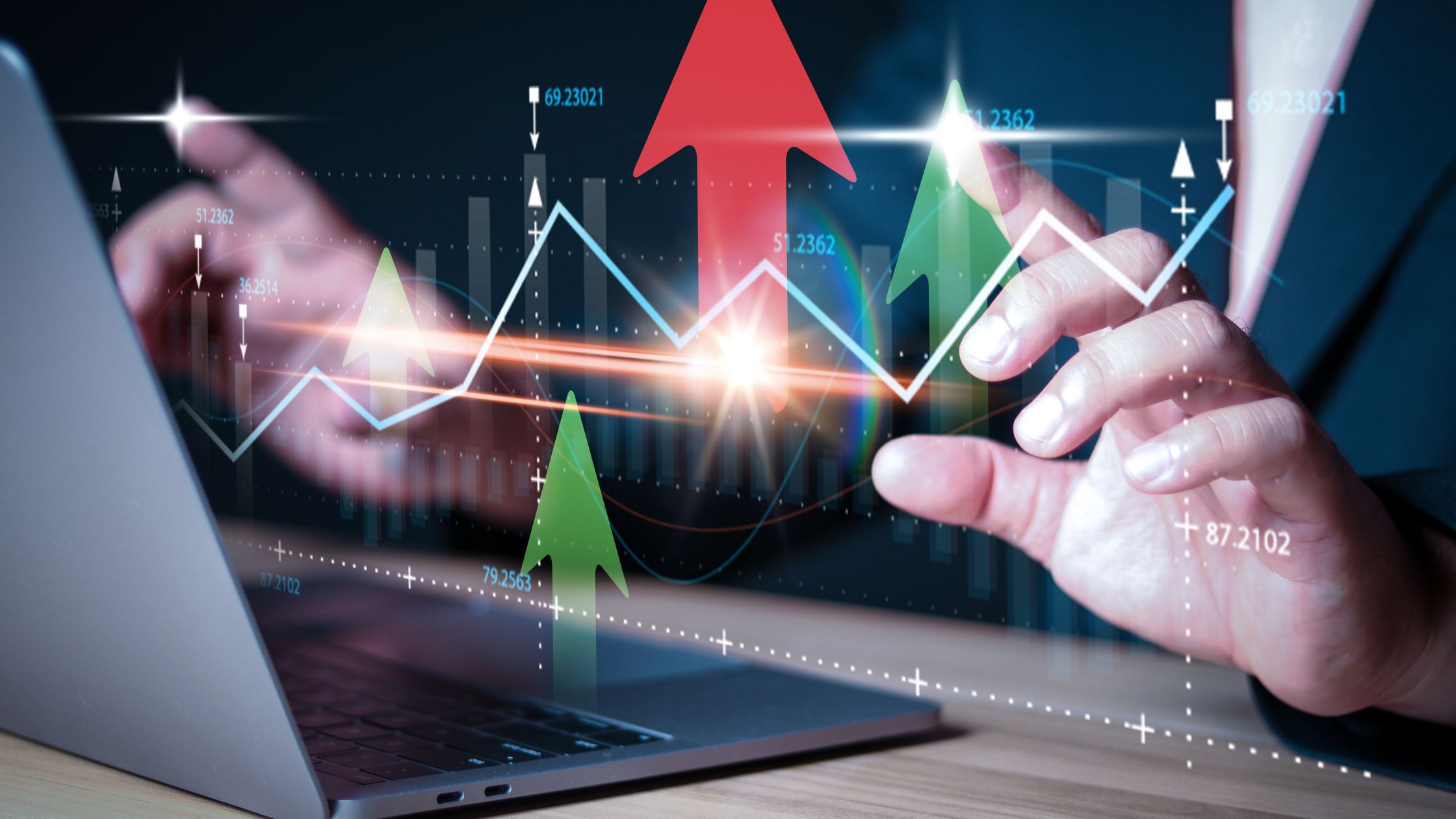
- by by Team Handson
- August 30, 2024
Comprehensive Credit Risk Assessment Using Advanced Machine Learning Techniques
1. Introduction
In the banking sector, accurately predicting credit risk is essential for minimizing financial loss and making informed lending decisions. This project aims to develop a sophisticated machine learning model to assess credit risk by integrating diverse datasets, applying advanced modeling techniques, and ensuring compliance with industry standards. The goal is to provide a nuanced understanding of customer creditworthiness and improve the bank's risk management strategies.
2. Objectives
-Primary Objective: To build a highly accurate machine learning model that predicts the likelihood of credit default by integrating customer, credit history, and loan performance data.
-Secondary Objectives:
- Conduct advanced feature engineering and selection to enhance model performance.
- Compare and optimize various machine learning algorithms, including ensemble methods and deep learning models.
- Implement a robust model evaluation framework with cross-validation and hyperparameter tuning.
- Develop an interactive dashboard for real-time credit risk assessment and visualization.
3. Problem Statement
Banks face the challenge of predicting credit default risk with high accuracy to mitigate potential losses. Traditional credit scoring models may not capture all relevant factors, leading to suboptimal decision-making. This project aims to improve the prediction of credit risk using advanced machine learning techniques, enhancing risk management and lending practices.
4. Data Requirements
The project will utilize a comprehensive dataset that includes customer demographics, credit history, and loan performance data. The dataset should be well-structured and include the following:
a. Customer Information Dataset (`customer_info.csv`)
- Variables:
- `CustomerID` (Integer): Unique identifier for the customer
- `Age` (Integer): Age of the customer
- `Gender` (Categorical): Gender of the customer (e.g., `Male`, `Female`)
- `MaritalStatus` (Categorical): Marital status (e.g., `Single`, `Married`, `Divorced`)
- `Income` (Numeric): Annual income of the customer
- `EmploymentStatus` (Categorical): Employment status (e.g., `Employed`, `Unemployed`, `Self-Employed`)
- `EducationLevel` (Categorical): Education level (e.g., `High School`, `Bachelor's`, `Master's`, `PhD`)
b. Credit History Dataset (`credit_history.csv`)
- Variables:
- `CustomerID` (Integer): Unique identifier for the customer
- `CreditScore` (Numeric): Credit score of the customer
- `LoanAmount` (Numeric): Amount of the loan
- `LoanTerm` (Numeric): Term of the loan in months
- `PreviousDefaults` (Integer): Number of previous credit defaults
- `CurrentBalance` (Numeric): Current balance of the customer
- `CreditUtilization` (Numeric): Ratio of credit used to credit available
c. Loan Performance Dataset (`loan_performance.csv`)
- Variables:
- `CustomerID` (Integer): Unique identifier for the customer
- `LoanID` (Integer): Unique identifier for the loan
- `LoanStatus` (Categorical): Status of the loan (`Default`, `Paid`, `In-Progress`)
- `PaymentHistory` (Categorical): Payment history (e.g., `Good`, `Poor`)
- `MonthlyPayment` (Numeric): Amount of the monthly payment
- `OverduePayments` (Integer): Number of overdue payments
5. Methodology
5.1 Data Preparation
- Data Integration: Merge datasets on `CustomerID` to create a unified view.
- Data Cleaning: Handle missing values, outliers, and data inconsistencies.
- Feature Engineering: Create new features (e.g., `Debt-to-Income Ratio`, `Loan-to-Value Ratio`) and perform transformations (e.g., scaling, encoding).
```python
import pandas as pd
from sklearn.preprocessing import LabelEncoder, StandardScaler
from sklearn.impute import SimpleImputer
# Load datasets
customer_info = pd.read_csv('customer_info.csv')
credit_history = pd.read_csv('credit_history.csv')
loan_performance = pd.read_csv('loan_performance.csv')
# Merge datasets
data = customer_info.merge(credit_history, on='CustomerID').merge(loan_performance, on='CustomerID')
# Handle missing values
imputer = SimpleImputer(strategy='median')
data.fillna(imputer.fit_transform(data), inplace=True)
# Feature Engineering
data['DebtToIncomeRatio'] = data['LoanAmount'] / data['Income']
data['LoanToValueRatio'] = data['LoanAmount'] / data['CurrentBalance']
# Encode categorical variables
label_encoder = LabelEncoder()
categorical_features = ['Gender', 'MaritalStatus', 'EmploymentStatus', 'EducationLevel', 'LoanStatus']
for feature in categorical_features:
data[feature] = label_encoder.fit_transform(data[feature])
# Scale features
scaler = StandardScaler()
data_scaled = pd.DataFrame(scaler.fit_transform(data.drop(['CustomerID', 'LoanID', 'LoanStatus'], axis=1)), columns=data.columns[2:])
```
5.2 Model Development and Optimization
- Exploratory Data Analysis (EDA): Perform advanced EDA to understand feature distributions and relationships.
- Model Selection: Implement and compare multiple machine learning algorithms, including:
- Random Forest
- Gradient Boosting Machines (GBM)
- XGBoost
- Neural Networks (Deep Learning)
- Hyperparameter Tuning: Use techniques like Grid Search and Random Search to optimize model parameters.
- Cross-Validation: Employ k-fold cross-validation to evaluate model performance.
```python
from sklearn.model_selection import train_test_split, GridSearchCV
from sklearn.ensemble import RandomForestClassifier
from sklearn.ensemble import GradientBoostingClassifier
from sklearn.neural_network import MLPClassifier
import xgboost as xgb
# Split data
X = data_scaled
y = data['LoanStatus']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Define models
models = {
'RandomForest': RandomForestClassifier(),
'GradientBoosting': GradientBoostingClassifier(),
'XGBoost': xgb.XGBClassifier(),
'NeuralNetwork': MLPClassifier()
}
# Hyperparameter tuning
param_grid = {
'RandomForest': {'n_estimators': [50, 100, 200], 'max_depth': [10, 20, 30]},
'GradientBoosting': {'n_estimators': [50, 100], 'learning_rate': [0.01, 0.1, 0.2]},
'XGBoost': {'n_estimators': [50, 100], 'max_depth': [3, 6, 9]},
'NeuralNetwork': {'hidden_layer_sizes': [(50,), (100,), (50, 50)], 'activation': ['relu', 'tanh']}
}
# Train and evaluate models
for model_name, model in models.items():
grid_search = GridSearchCV(model, param_grid[model_name], cv=5, scoring='accuracy', n_jobs=-1)
grid_search.fit(X_train, y_train)
best_model = grid_search.best_estimator_
print(f"{model_name} Best Parameters: {grid_search.best_params_}")
print(f"{model_name} Accuracy: {best_model.score(X_test, y_test)}")
```
5.3 Model Deployment and Dashboard Development
-Model Deployment: Deploy the best-performing model using a REST API framework like Flask or FastAPI for real-time predictions.
-Dashboard Development: Create a comprehensive dashboard for visualizing credit risk predictions and customer insights using tools like Tableau or Power BI.
```python
# Example Flask API for deployment
from flask import Flask, request, jsonify
import pickle
app = Flask(__name__)
# Load model
with open('best_model.pkl', 'rb') as file:
model = pickle.load(file)
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json(force=True)
prediction = model.predict([data['features']])
return jsonify({'prediction': int(prediction[0])})
if __name__ == '__main__':
app.run(port=5000)
```
**6. Expected Outcomes**
- High-Accuracy Predictive Model: A machine learning model with high predictive accuracy for credit risk, leading to better-informed lending decisions.
- Detailed Insights: An interactive dashboard providing real-time visualizations and insights into credit risk and customer profiles.
- Enhanced Risk Management: Improved ability to assess and manage credit risk, reducing financial exposure and enhancing portfolio performance.
7. Tools and Technologies
- Programming Languages: Python
- Libraries: pandas, scikit-learn, xgboost, matplotlib, seaborn, Flask
- Tools: Jupyter Notebook for development, Tableau or Power BI for dashboard creation, REST API frameworks for deployment
8. Timeline
- Week 1-2:Data Collection and Integration
- Week 3-4: Data Cleaning, Feature Engineering, and EDA
- Week 5-6: Model Development and Hyperparameter Tuning
- Week 7-8: Model Evaluation and Optimization
- Week 9-10: Deployment and Dashboard Development
- Week 11-12: Final
Report Preparation and Presentation
This project provides a robust framework for predicting credit risk in the banking industry using advanced machine learning techniques. By integrating diverse datasets and applying sophisticated modeling approaches, the project aims to enhance risk assessment, improve lending decisions, and offer valuable insights into customer creditworthiness.
This enhanced project synopsis outlines a complex and real-time approach to credit risk prediction, incorporating advanced techniques and comprehensive methodologies relevant to the banking industry.
Here's a detailed project report for a machine learning project aimed at predicting credit risk in the banking industry. The report includes executable code for each stage of the project, from data preparation to model deployment.
**Project Report: Comprehensive Credit Risk Assessment Using Advanced Machine Learning Techniques**